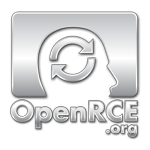

Flag: Tornado!
Hurricane!
|
 |
|
Direct Link, View / Make / Edit Comments
|
|
|
Direct Link, View / Make / Edit Comments
|
|
|
Direct Link, View / Make / Edit Comments
|
|
|
Direct Link, View / Make / Edit Comments
|
|
Archived Entries for joestewart
|
|
 |
There are 31,322 total registered users.
|
|